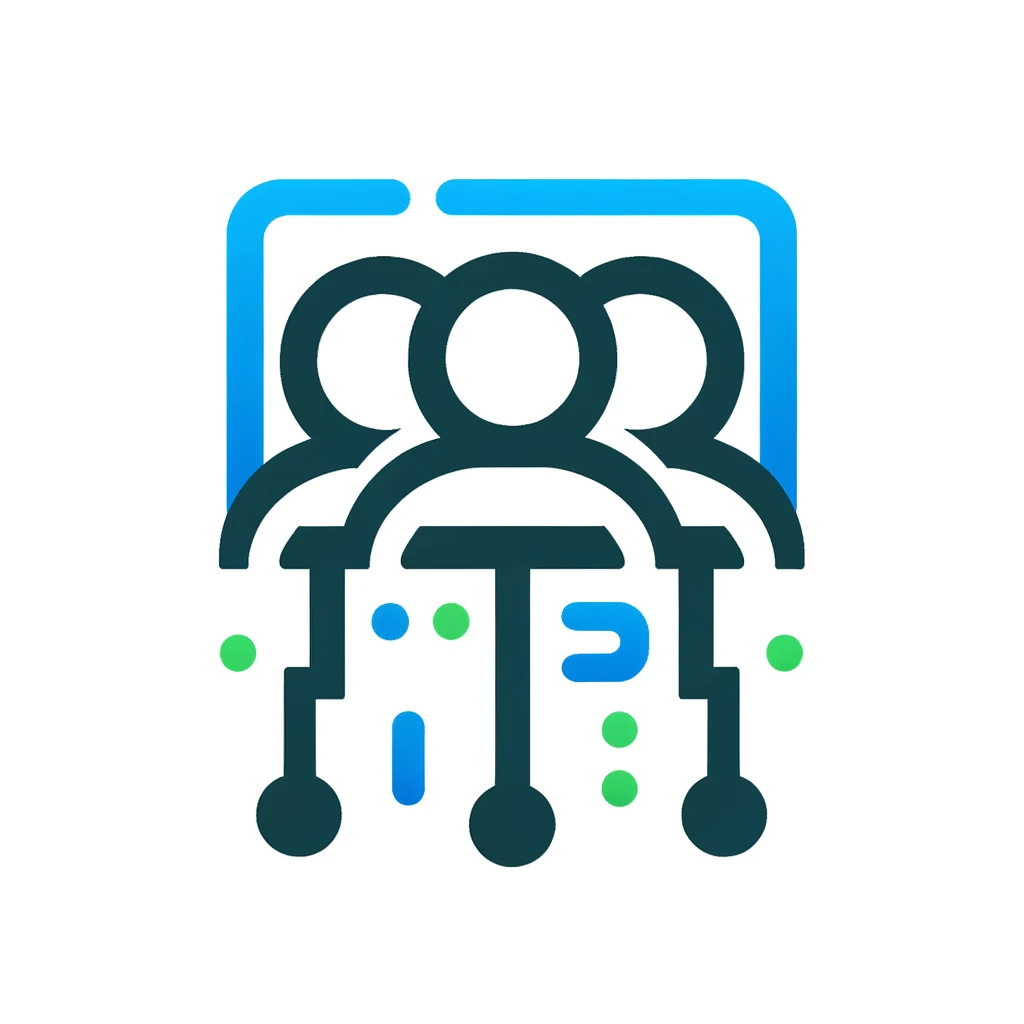
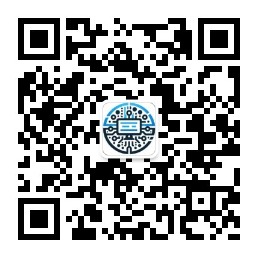
Call OpenAI API by PHP
2023-12-14
1. Setup OpenAI API key.
- $openai_api_key = 'sk-xxxxxx';
2. Setup call url, we use 'chat completions' for example.
- $url = 'https://api.openai.com/v1/chat/completions';
You can referer to OpenAI API's endpoints for a complete list of call urls at the following address: https://platform.openai.com/docs/guides/text-generation
3. Setup authentication info for the call.
- $header = "Content-Type: application/json\nAuthorization: Bearer $openai_api_key ";
4. Appoint the model you want to call.
- $requestObj = new stdClass();
- $requestObj->model = 'gpt-4-1106-preview';
You can get a full model list from OpenAI API's document at the folling address: https://platform.openai.com/docs/models
5. Optional: setup return results format to JSON.
For easier use of the return results, you can setup the API to return results in JSON format. If you don't need JSON format, you can skip this step. Important: if you setup the return results in JSON format, you MUST also talk to the API to return in JSON format explicitly in the following step.
- $response_format = new stdClass();
- $response_format->type = 'json_object';
- $requestObj->response_format = $response_format;
6. Setup the system role of the call.
- $messages = [];
- $message = new stdClass();
- $message->role = 'system';
- $message->content = 'You are a poet';
- $messages[] = $message;
7. Setup user messages to pass to the call.
- $message = new stdClass();
- $message->role = 'user';
- $message->content = 'Please write a poem about regress. Please return results in JSON format.';
- $messages[] = $message;
- $requestObj->messages = $messages;
Important: if you setup the return results in JSON format in the previous step, you MUST also set the return type to JSON explicitly here.
If the message is too long, you can split it into several lines as following, for better readable of the code.
- $message = new stdClass();
- $message->role = 'user';
- $message->content = <<<EOD
- Please write a poem about regress.
- Please return results in JSON format.
- EOD;
- $messages[] = $message;
- $requestObj->messages = $messages;
8. Prepare the request contents by encoding the previous object.
- $request = json_encode($requestObj);
9. Create a request object.
- $opts = array(
- 'http' => [
- 'method' => 'POST',
- 'header' => $header,
- 'content' => $request,
- 'timeout' => 300
- ]
- );
- $context = stream_context_create($opts);
For some reasons, your server which you will use to call the API, may lost CA certificates, or CA certificates are not newest. This will result in failure in SSL verify when make a call. In this situation, you should update the CA certificates, or just skip certificate verify. But skip certificate verify is not recommended.
- $opts = array(
- 'http' => [
- 'method' => 'POST',
- 'header' => $header,
- 'content' => $request,
- 'timeout' => 300
- ],
- 'ssl' => [
- 'verify_peer' => false,
- 'verify_peer_name' => false
- ]
- );
- $context = stream_context_create($opts);
10. Send the request top OpenAI API.
- $response = @file_get_contents($url, false, $context);
11. Handle any error that may occupied in the request. The following is a sample example.
- if($response === false){
- var_dump($http_response_header);
- die();
- }
If error occupied, please check the error messages carefully. For example, if the request format is wrong, or the model name is incorrect, the OpenAI server may return HTTP 400 error message, but it's not meaning the OpenAI server is error, instead, it's your error.
12. Decode the result for your latter uses.
- $responseObj = json_decode($response);
- echo $responseObj->choices[0]->message->content;
The full return result can be refered at OpenAI's documents at the following address: https://platform.openai.com/docs/introduction. Or, you can just execute var_dump($responseObj) to get all returned properties.
13. The full code is as following.
- $openai_api_key = 'sk-xxxxxx';
- $url = 'https://api.openai.com/v1/chat/completions';
- $header = "Content-Type: application/json Authorization: Bearer $openai_api_key ";
- $requestObj = new stdClass();
- $requestObj->model = 'gpt-4-1106-preview';
- $response_format = new stdClass();
- $response_format->type = 'json_object';
- $requestObj->response_format = $response_format;
- $messages = [];
- $message = new stdClass();
- $message->role = 'system';
- $message->content = 'You are a poet';
- $messages[] = $message;
- $message = new stdClass();
- $message->role = 'user';
- $message->content = <<<EOD
- Please write a poem about regress.
- Please return results in JSON format.
- EOD;
- $messages[] = $message;
- $requestObj->messages = $messages;
- $request = json_encode($requestObj);
- $opts = array(
- 'http' => [
- 'method' => 'POST',
- 'header' => $header,
- 'content' => $request,
- 'timeout' => 300
- ],
- 'ssl' => [
- 'verify_peer' => false,
- 'verify_peer_name' => false
- ]
- );
- $context = stream_context_create($opts);
- $response = @file_get_contents($url, false, $context);
- if($response === false){
- var_dump($http_response_header);
- die();
- }
- $responseObj = json_decode($response);
- echo $responseObj->choices[0]->message->content;
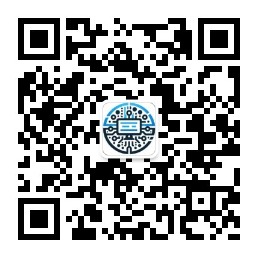
Follow us at WeChat to get more info