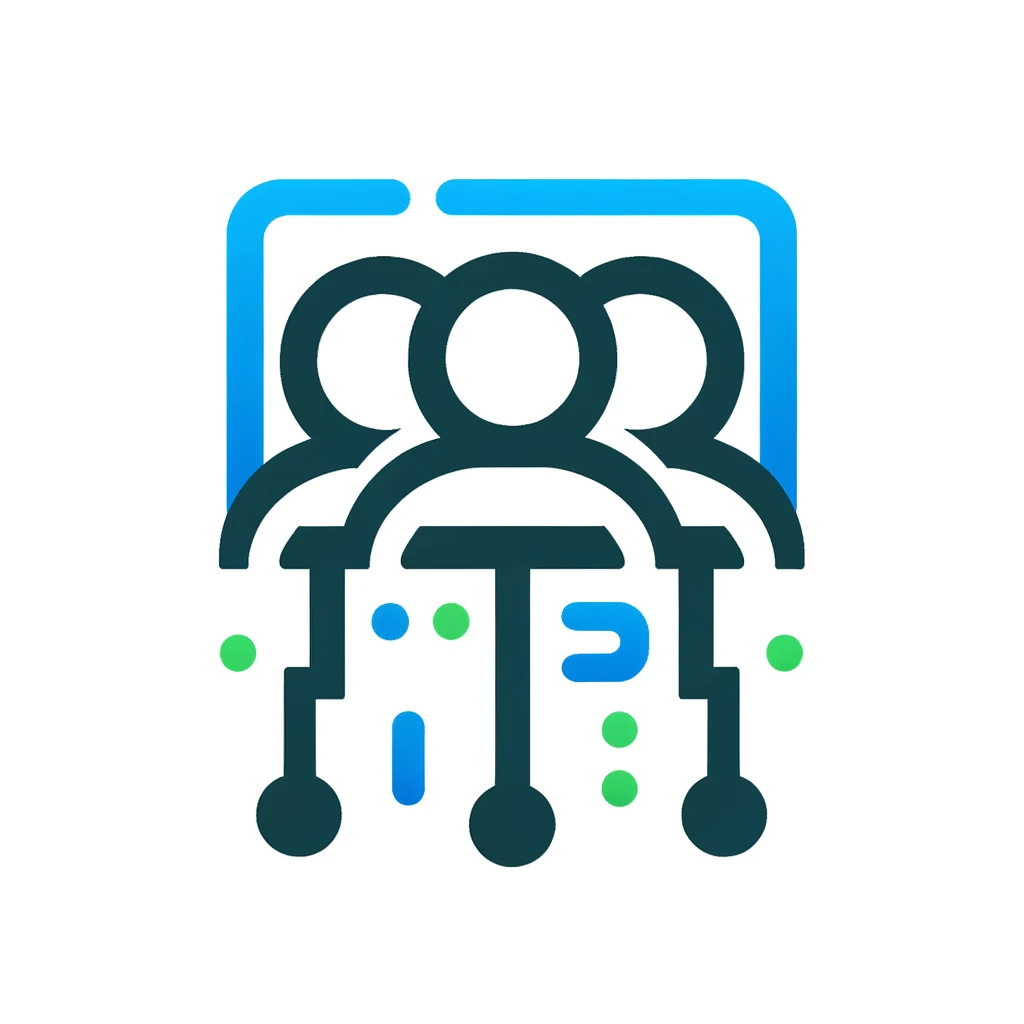
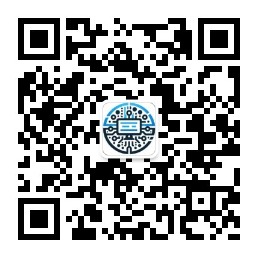
Security Coding using PHP -- Write Safe Code
14. Buffer Overflow Issues Caused by Insecure Code
2024-07-10
Buffer overflow is a common security vulnerability where an attacker writes more data to a buffer than it can hold, thereby overwriting adjacent memory regions. This can lead to attackers executing arbitrary code, accessing sensitive data, or causing system instability. This article will introduce the background knowledge of buffer overflow, provide examples of related security issues, and discuss how to prevent these problems.
Questions
Question 1. What is a buffer overflow?
Question 2. How can attackers exploit buffer overflow vulnerabilities?
Question 3. List two methods to prevent buffer overflow.
Units
1. Introduction to Background Knowledge
2. Example 1: Simple String Copy
3. Example 2: File Read Operation
4. Example 3: Unsafe Array Operation
5. How to Prevent
6. Additional Knowledge
1. Introduction to Background Knowledge
Buffer overflow occurs when a program writes more data to a buffer than it can hold, causing adjacent memory regions' data to be overwritten. This situation typically occurs in languages like C and C++, but can also appear in other languages. Attackers can exploit buffer overflow to overwrite important data in memory, leading to arbitrary code execution, access to sensitive information, or program crashes.
2. Example 1: Simple String Copy
Problematic Code Example:
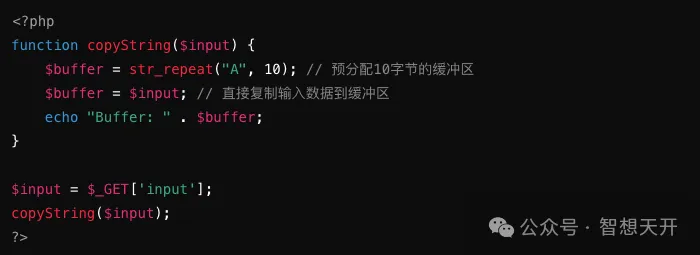
Attackers can overflow the buffer by passing a long string:
http://example.com/vulnerable.php?input=AAAAAAAAAAAAAAAAAAAAAAAA
The attacker overflows the buffer by passing a long string, potentially overwriting other important data in memory.
3. Example 2: File Read Operation
Problematic Code Example:
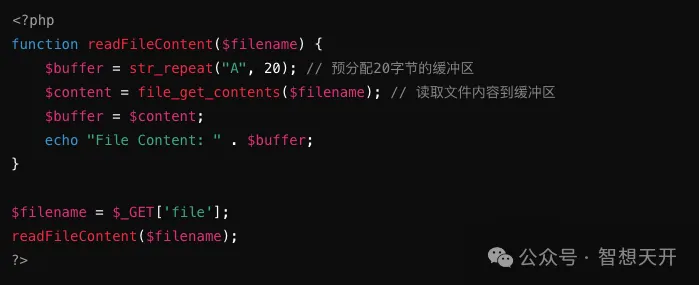
Attackers can overflow the buffer by passing a file path:
http://example.com/vulnerable.php?file=/etc/passwd
The attacker overflows the buffer by reading a large file, potentially overwriting other important data in memory.
4. Example 3: Unsafe Array Operation
Problematic Code Example:
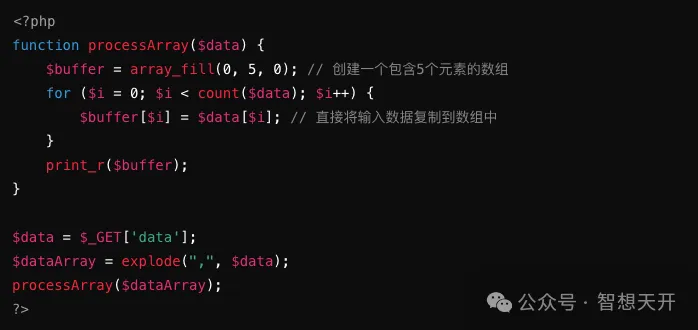
Attackers can overflow the buffer by passing a long array:
http://example.com/vulnerable.php?data=1,2,3,4,5,6,7,8,9
The attacker overflows the buffer by passing a long array, potentially overwriting other important data in memory.
5. How to Prevent
5.1. Fix for Example 1: Simple String Copy
Fixed Secure Code:
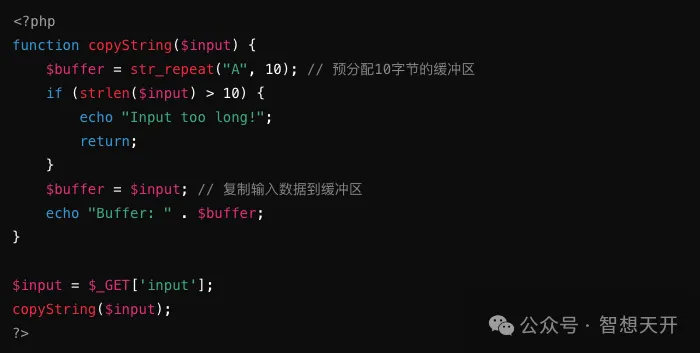
By checking the length of the input data, it prevents the buffer from being overflowed by a long string, avoiding buffer overflow.
5.2. Fix for Example 2: File Read Operation
Fixed Secure Code:
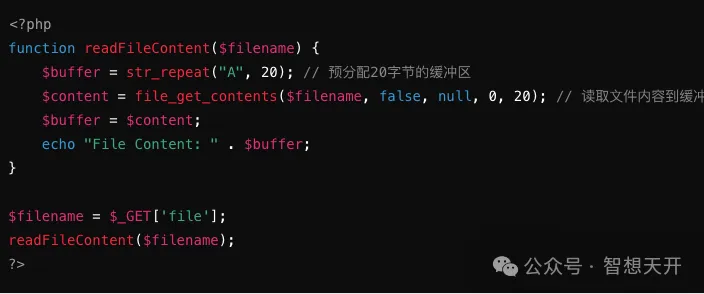
By limiting the length of the file content read, it prevents the buffer from being overflowed by a large file, avoiding buffer overflow.
5.3. Fix for Example 3: Unsafe Array Operation
Fixed Secure Code:
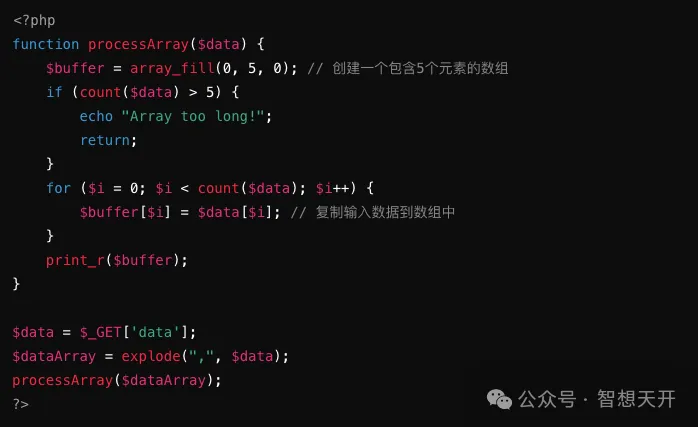
By checking the length of the input data, it prevents the buffer from being overflowed by a long array, avoiding buffer overflow.
6. Additional Knowledge
Input Validation and Boundary Checking: Always perform input validation and boundary checking when handling external inputs to ensure the data does not exceed the expected range.
Secure Coding Practices: Understand and follow best practices for secure coding, such as using secure functions instead of insecure ones.
Use Secure Libraries and Frameworks: Choose security-audited libraries and frameworks that typically have better protection measures.
Deep Learning on Security: Read OWASP guidelines to learn more about buffer overflow and other security issues.
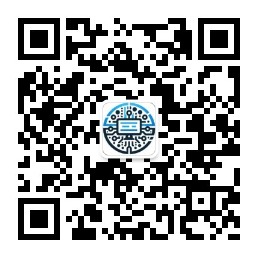
Follow us at WeChat to get more info