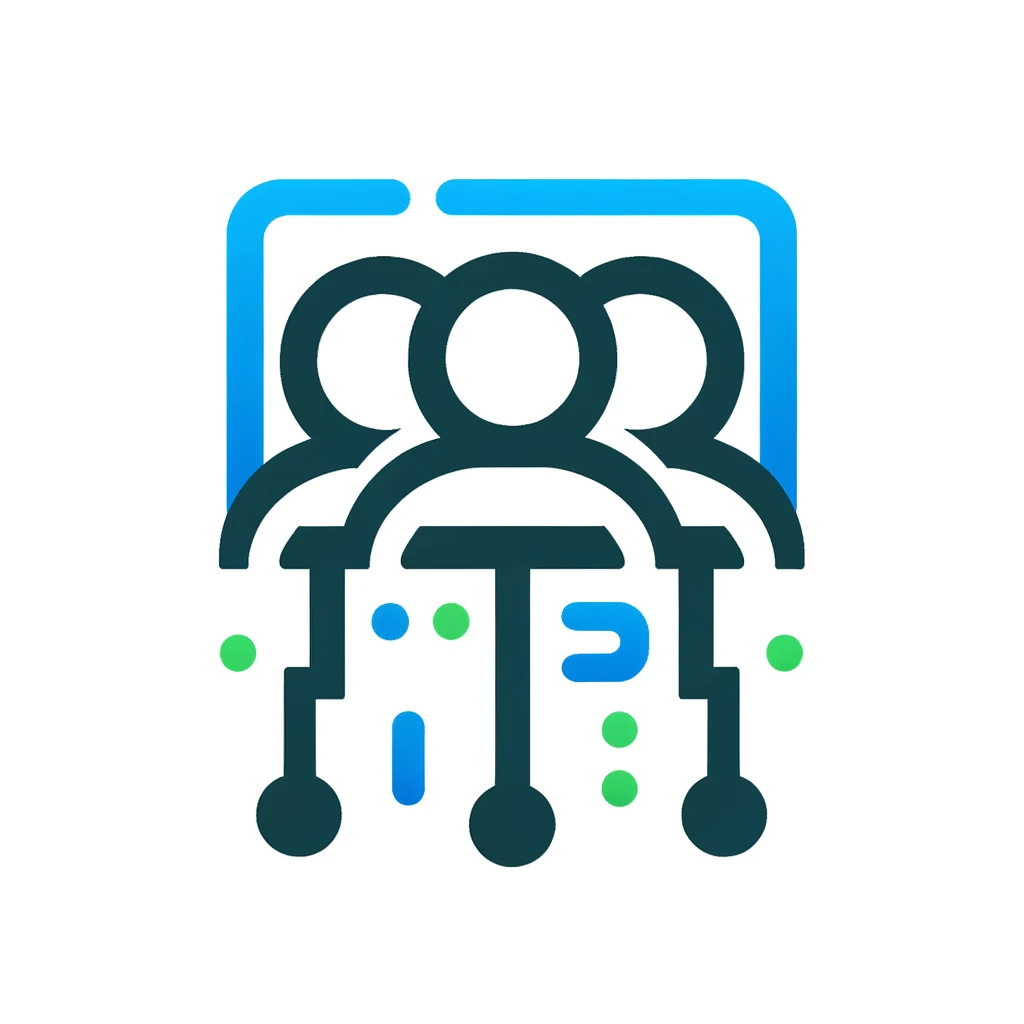
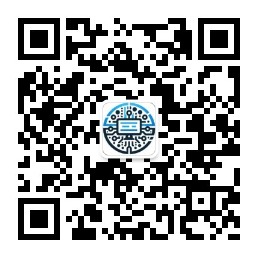
Security Coding using PHP -- Write Safe Code
11. Unsafe Deserialization
2024-07-05
Deserialization is the process of converting stored or transmitted data back into objects or data structures within a program. However, insecure deserialization can lead to severe security issues, especially when dealing with data from untrusted sources. This article will introduce the background knowledge of deserialization, provide examples of security issues caused by incorrect deserialization using PHP, and discuss how to prevent these issues.
Questions
Question 1. What is deserialization, and why is it necessary to be especially careful when handling untrusted data?
Question 2. How can deserialization vulnerabilities be exploited?
Question 3. List two methods to prevent insecure deserialization.
Units
1. Introduction to Background Knowledge
2. Example 1: PHP Object Injection
3. Example 2: PHP Magic Method Injection
4. Example 3: PHP Reflection Object Injection
5. Example 4: PHP Command Execution
6. How to Prevent
7. Additional Knowledge
1. Introduction to Background Knowledge
Deserialization is the process of converting serialized data (usually in binary or text format) back into original objects. Serialization and deserialization are widely used in data storage, remote communication, and cross-language data exchange. However, insecure deserialization can allow attackers to execute arbitrary code, access sensitive data, or disrupt system stability.
2. Example 1: PHP Object Injection
Problematic Code Example:
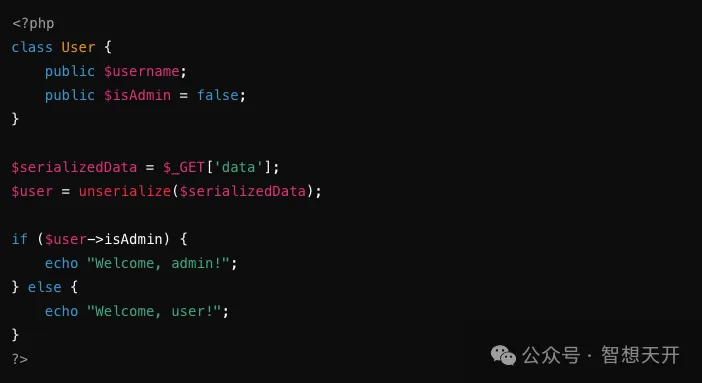
An attacker can pass malicious serialized data to escalate privileges. For example, an attacker constructs such attack code:

By passing the malicious serialized data using the following url, the attacker tricks the system into thinking they are an admin user, gaining unauthorized access to admin functionalities.
http://example.com/vulnerable.php?data=0%3A4%3A%22User%22%3A2%3A%7Bs%3A8%3A%22username%22%3Bs%3A5%3A%22guest%22%3Bs%3A7%3A%22isAdmin%22%3Bb%3A1%3B%7D
3. Example 2: PHP Magic Method Injection
Problematic Code Example:
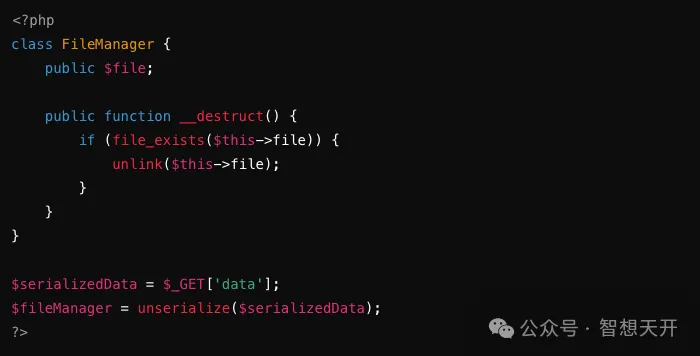
An attacker can use deserialized objects to delete arbitrary files. For example, an attacker constructs such attack code:

The attacker manages to delete the /etc/passwd file by the following url, potentially causing system instability or denial of service.
http://example.com/vulnerable.php?data=0%3A10%3A%22FileManager%22%3A1%3A%7Bs%3A4%3A%22file%22%3Bs%3A12%3A%22%2Fetc%2Fpasswd%22%3B%7D
4. Example 3: PHP Reflection Object Injection
Problematic Code Example:
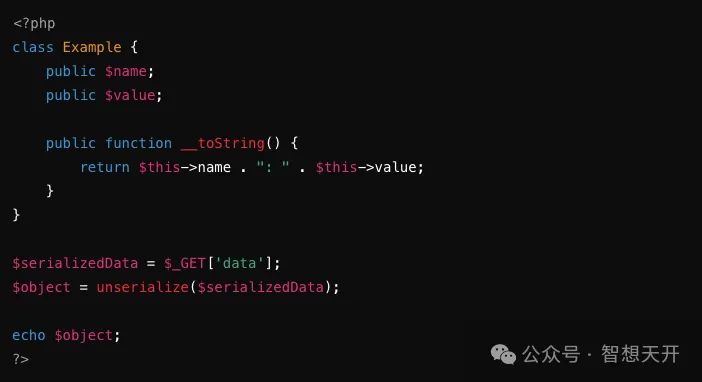
An attacker can inject malicious code through reflection object. For example, an attacker constructs such attack code:

By injecting a command using the following url, the attacker can execute arbitrary system commands, potentially leading to unauthorized access to system files and data.
http://example.com/vulnerable.php?data=0%3A8%3A%22Example%22%3A2%3A%7Bs%3A4%3A%22name%22%3Bs%3A6%3A%22system%22%3Bs%3A5%3A%22value%22%3Bs%3A12%3A%22ls%20%2Fvar%2Fwww%22%3B%7D
5. Example 4: PHP Command Execution
Problematic Code Example:
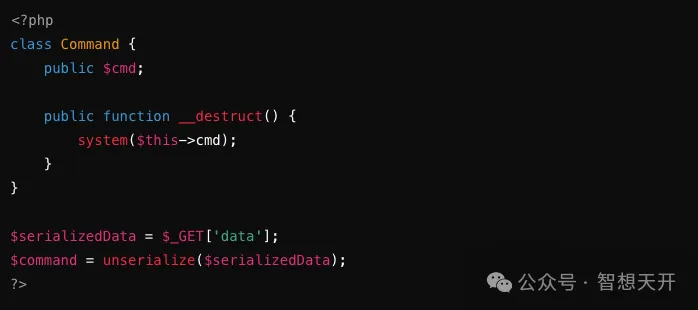
An attacker can use deserialized objects to execute arbitrary commands. For example, an attacker constructs such attack code:

The attacker executes a command to delete the /var directory with the following url, potentially causing significant data loss and system disruption.
http://example.com/vulnerable.php?data=0%3A7%3A%22Command%22%3A1%3A%7Bs%3A3%3A%22cmd%22%3Bs%3A12%3A%22rm%20-rf%20%2Fvar%22%3B%7D
6. How to Prevent
In PHP, when using the unserialize function, you can pass an options array to restrict the allowed classes for deserialization, effectively preventing security issues caused by untrusted data. For example, the ['allowed_classes' => false] option ensures that only simple data types can be deserialized, excluding any class instances. This method reduces the potential attack surface and makes the deserialization process more secure.
6.1. Fix for Example 1: PHP Object Injection
Fixed Secure Code:
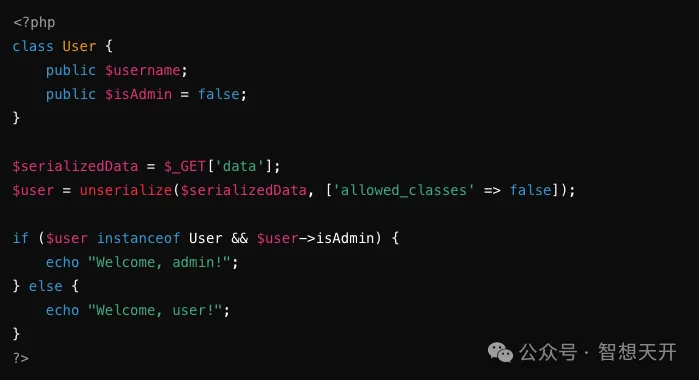
Since the unserialize function restricts the allowed classes, malicious data cannot create a User class instance, causing the attack to fail.
6.2. Fix for Example 2: PHP Magic Method Injection
Fixed Secure Code:
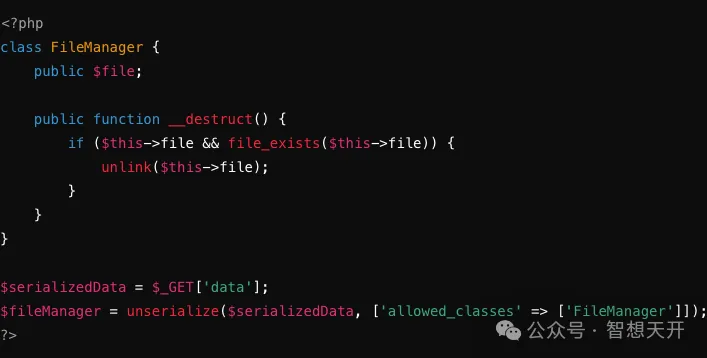
Since the unserialize function restricts to only allowing the FileManager class, malicious data with non-FileManager class instances cannot be deserialized, avoiding the risk of file deletion.
6.3. Fix for Example 3: PHP Reflection Object Injection
Fixed Secure Code:
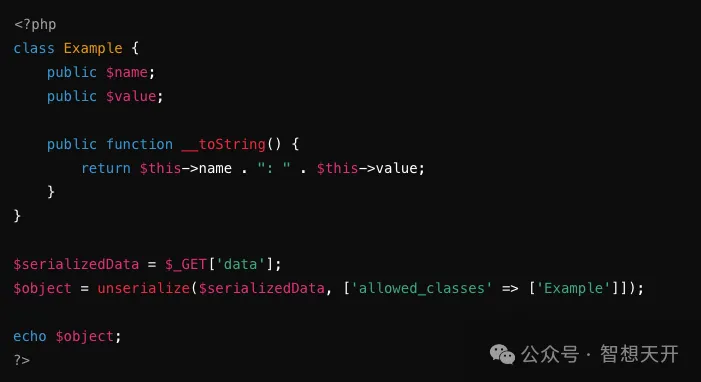
Since the unserialize function restricts to only allowing the Example class, malicious data with other class instances cannot be deserialized, avoiding the execution of malicious commands.
6.4. Fix for Example 4: PHP Command Execution
Fixed Secure Code:
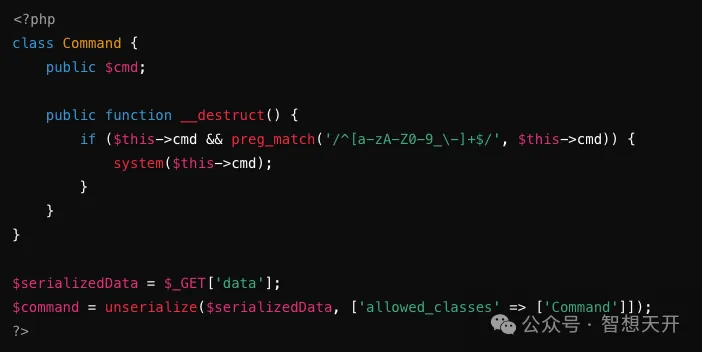
Since the unserialize function restricts to only allowing the Command class and checks the command with a regex pattern, malicious commands cannot be executed, avoiding the risk of system disruption.
7. Additional Knowledge
Alternatives to Serialization and Deserialization: Learn to use safer data formats such as JSON or XML for data transmission and storage.
Input Validation and Output Encoding: Strengthen input validation and output encoding to prevent injection attacks.
Use Secure Libraries and Frameworks:Choose libraries and frameworks that have undergone security audits, as they typically have better protection measures.
Deep Learning on Security: Read OWASP guidelines to learn more about deserialization vulnerabilities and other security issues.
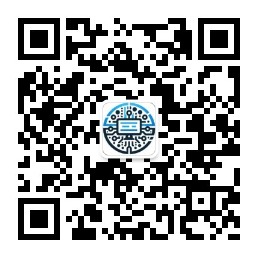
Follow us at WeChat to get more info