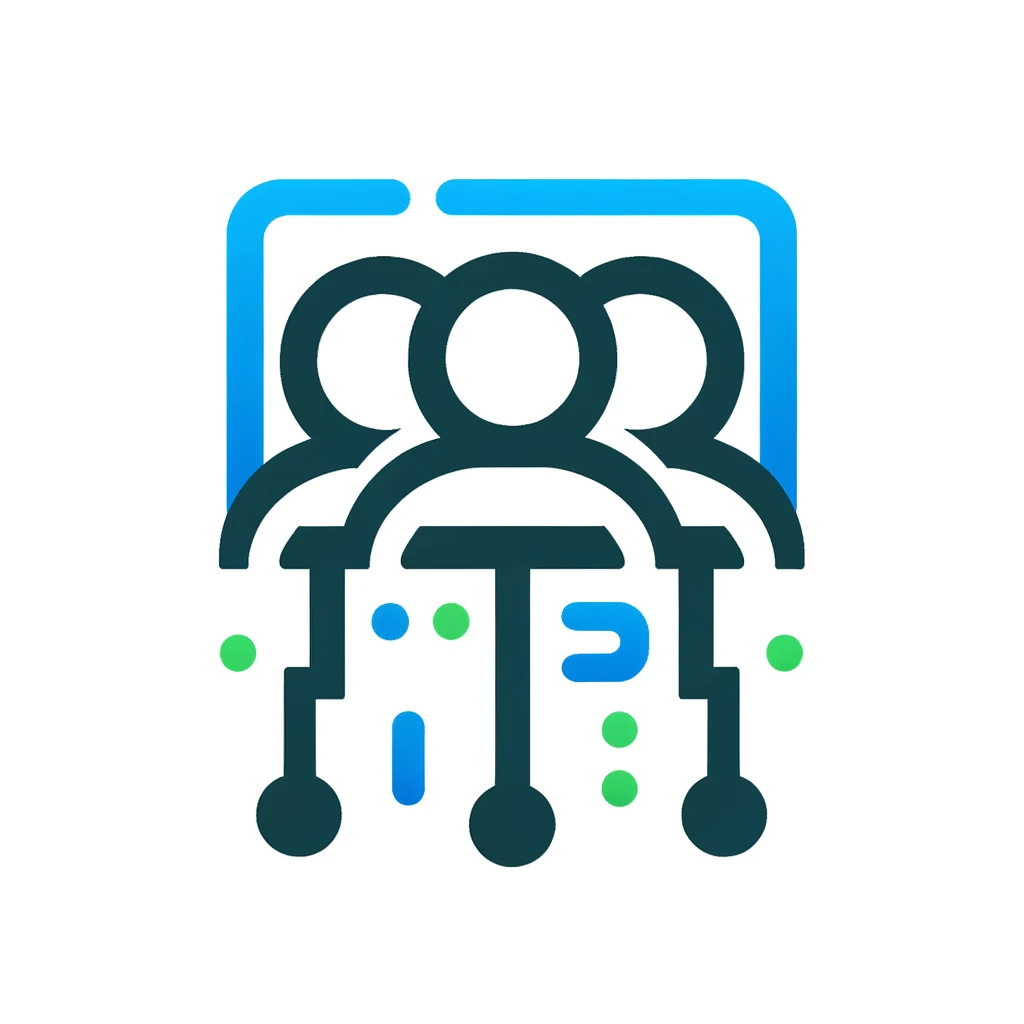
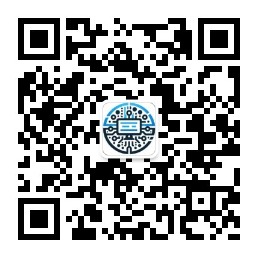
Common Code Issues and Their Solutions
2024-07-04
When writing and maintaining code, developers often encounter various issues. This article will describe some common code issues and provide problematic code examples along with their solutions using PHP as an example.
1. Duplicate code
Using the same or similar code in multiple places. This increases the difficulty of maintenance.
Problematic code example:
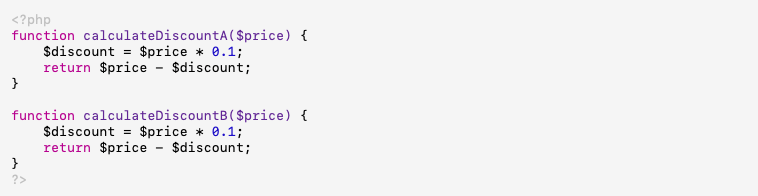
Solution example:
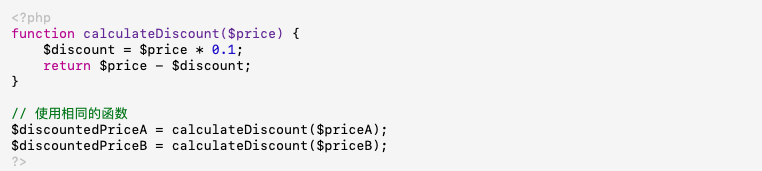
2. Long functions
The function is too long and does too many things, making it difficult to understand and maintain.
Problematic code example:
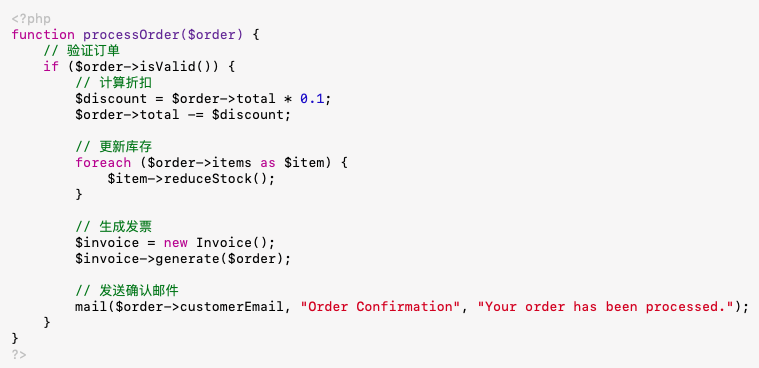
Solution example:
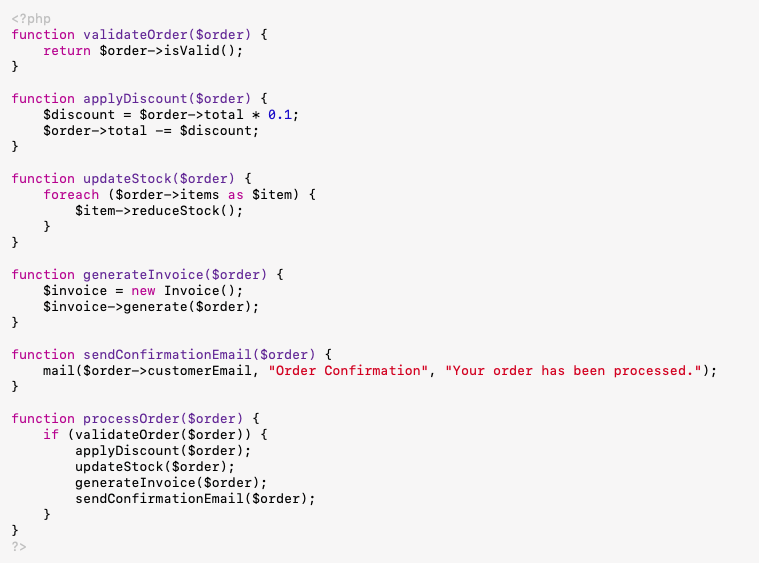
3. Large classes
A class takes on too many responsibilities, containing too many variables and methods. This violates the Single Responsibility Principle.
Problematic code example:
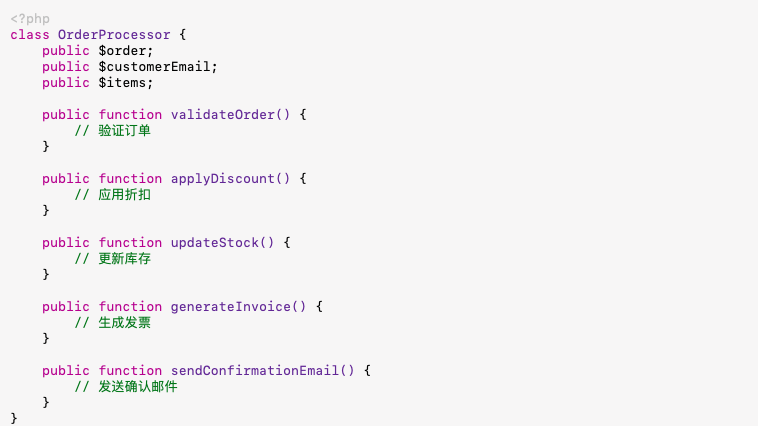
Solution example:
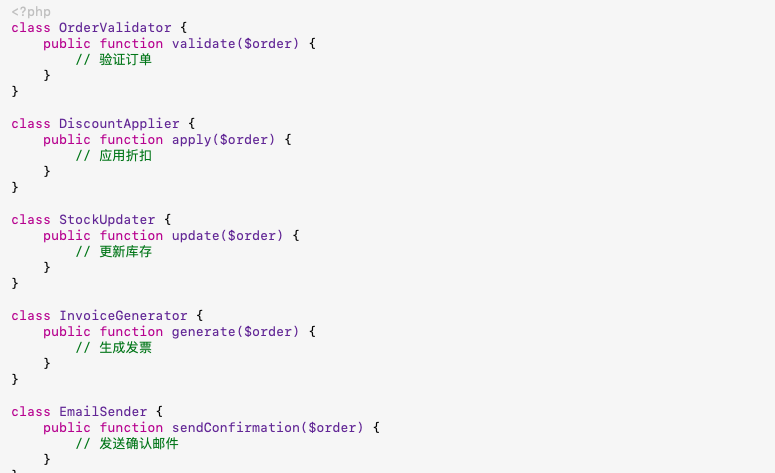
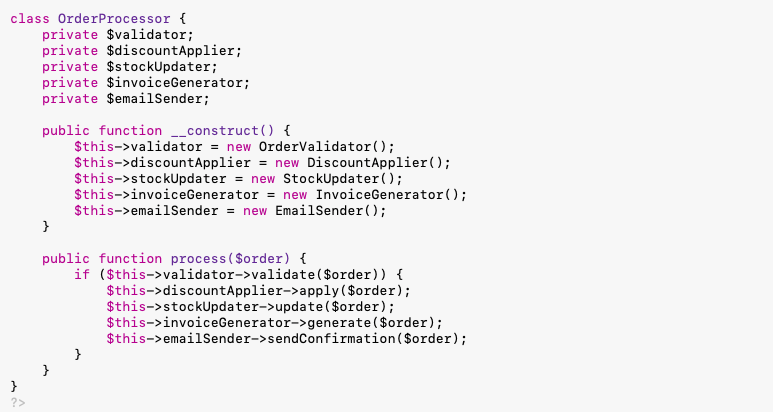
4. Long parameter lists
The parameter list of a function or method is too long, making it difficult to understand and use.
Problematic code example:

Solution example:
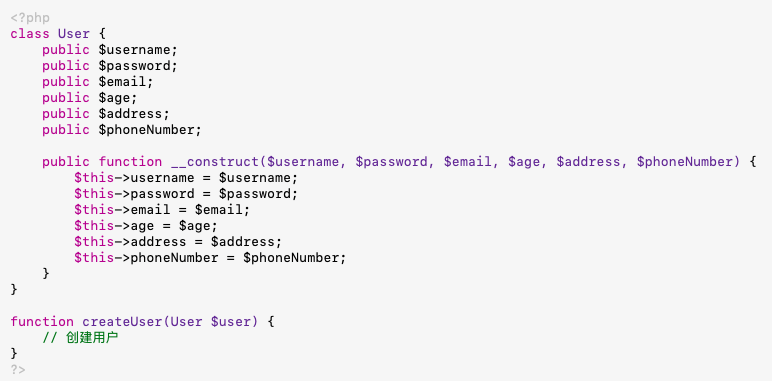
5. Divergent change
A module often changes for different reasons, violating the Single Responsibility Principle.
Problematic code example:
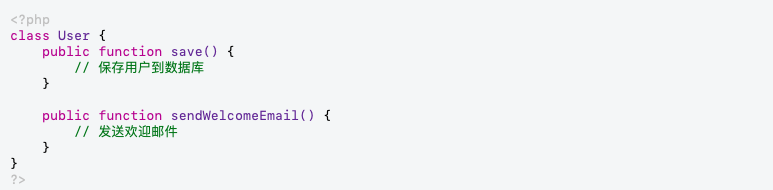
Solution example:
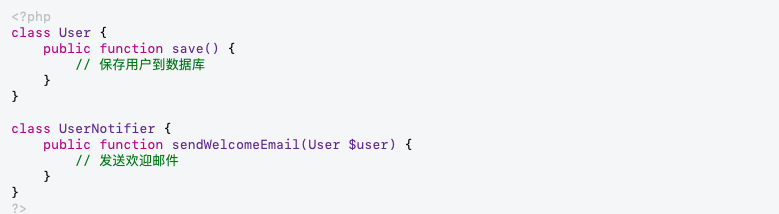
6. Shotgun surgery
Every time a feature is modified, changes need to be made in many different places.
Problematic code example:
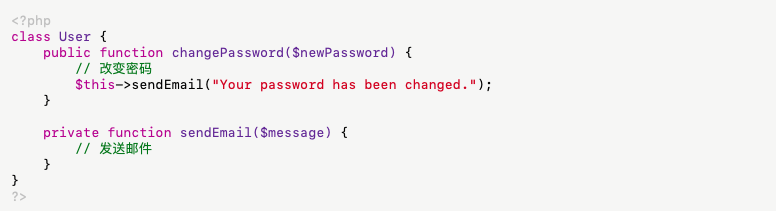
Solution example:
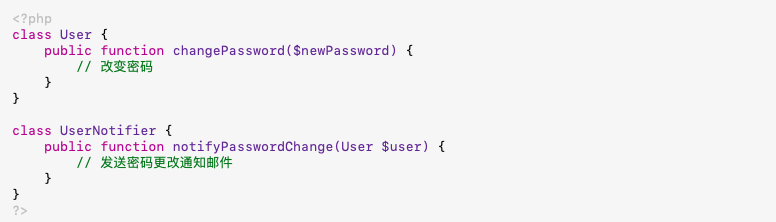
7. Feature envy
A class overly depends on the implementation details of another class, violating the encapsulation principle.
Problematic code example:
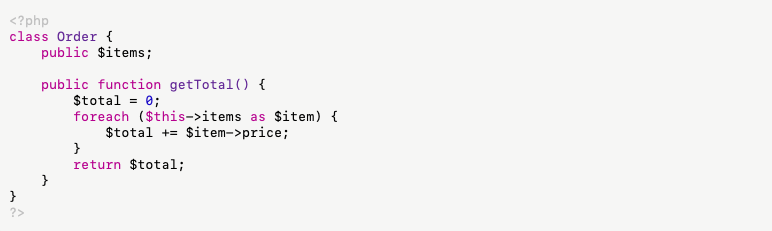
Solution example:
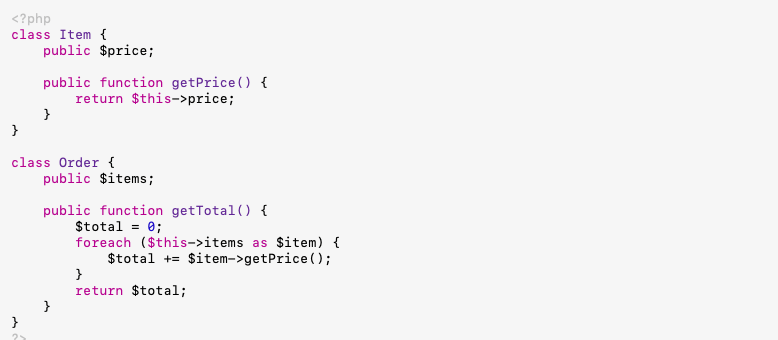
8. Data clumps
Too many basic data types are gathered together, forming complex structures that are difficult to maintain.
Problematic code example:

Solution example:
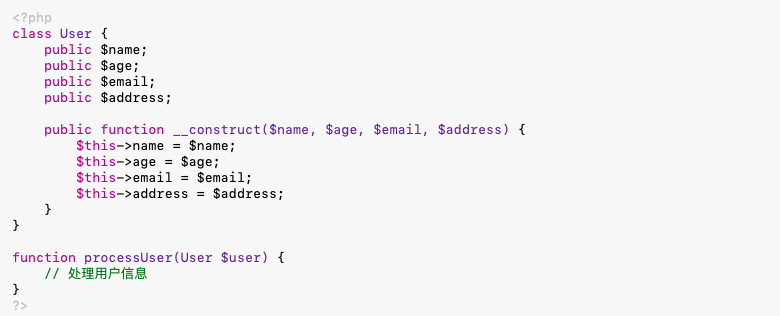
9. Primitive obsession
Overly relying on basic data types instead of using more suitable classes or data structures.
Problematic code example:
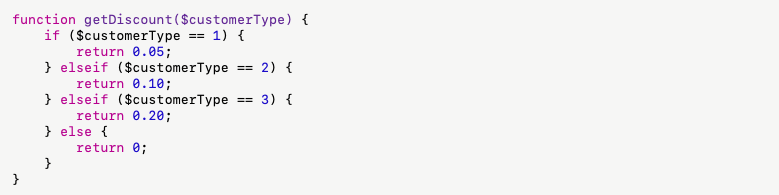
Solution example:
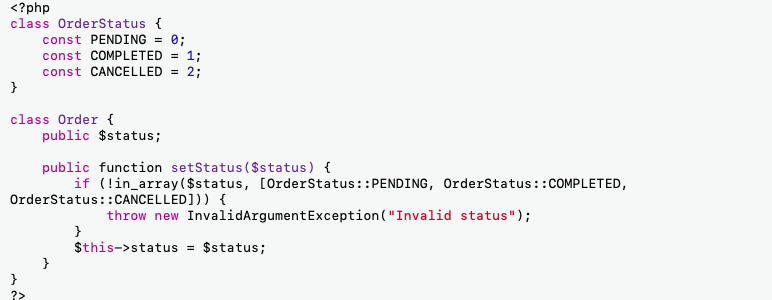
10. Repeated switch statements
Using the same switch statement multiple times to handle different values of the same variable.
Problematic code example:
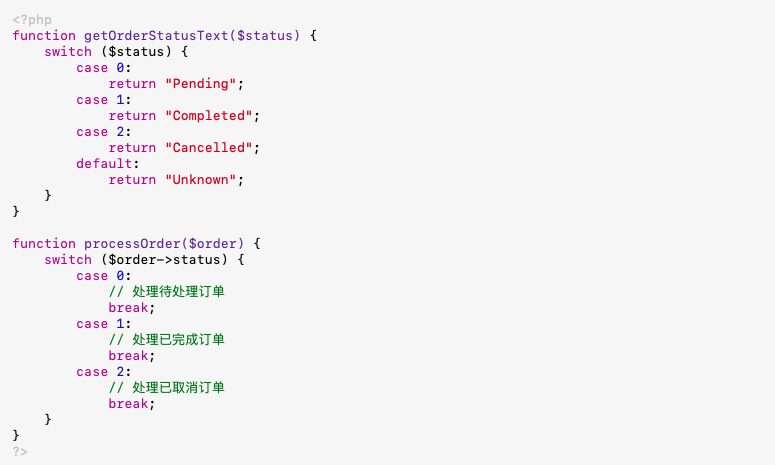
Solution example:
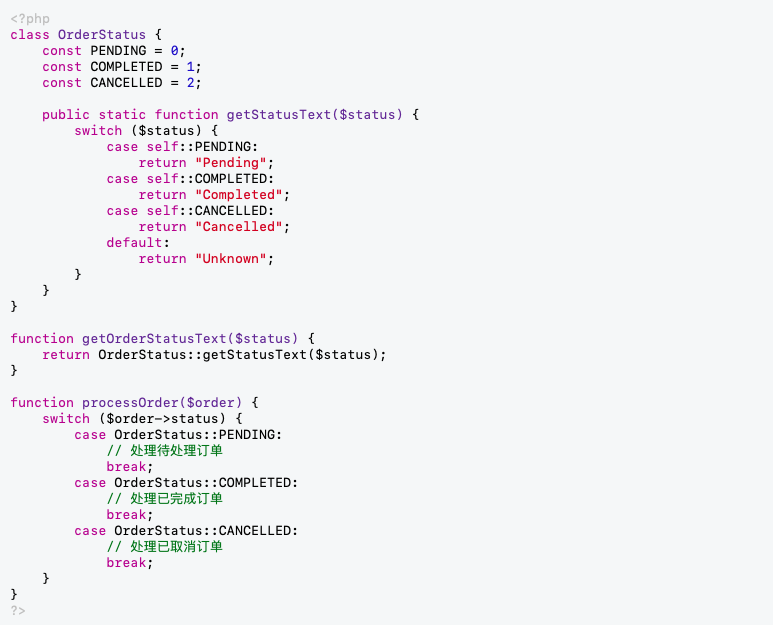
11. Lazy class/Redundant class
The class has too few functionalities to justify its existence, adding unnecessary complexity.
Problematic code example:

Solution example:

12. Over-engineering
The design and implementation are overly complex, exceeding current requirements.
Problematic code example:
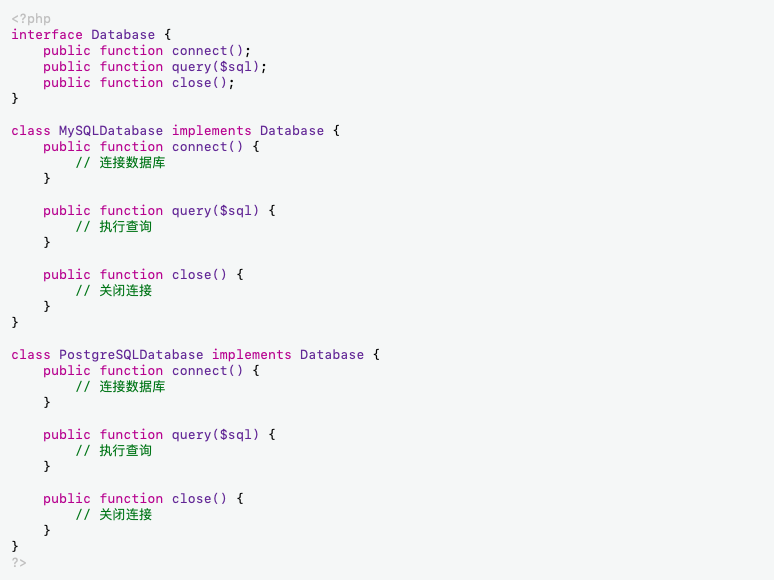
Solution example:
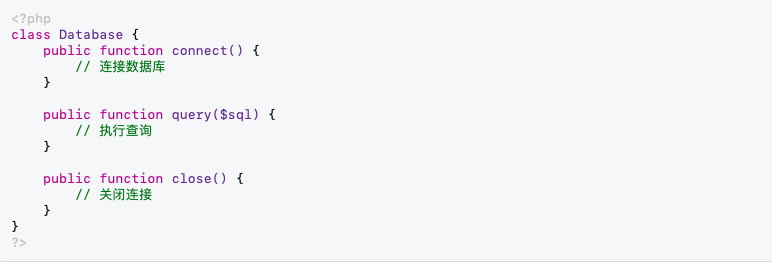
13. Temporary fields
Some fields in the class are only useful in specific situations, increasing the class's complexity.
Problematic code example:
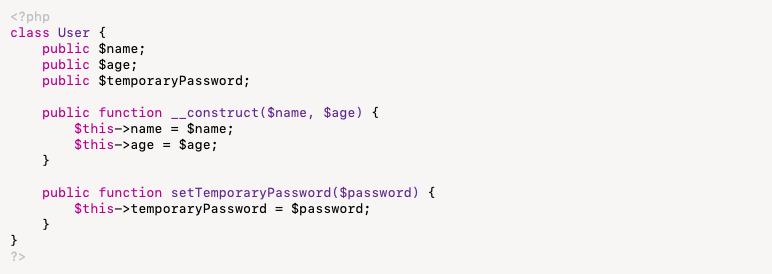
Solution example:
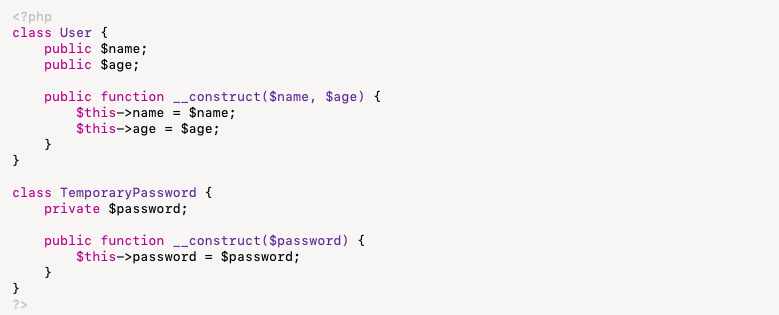
14. Message chain
Objects call methods of other objects through a long chain, increasing dependency and coupling.
Problematic code example:

Solution example:
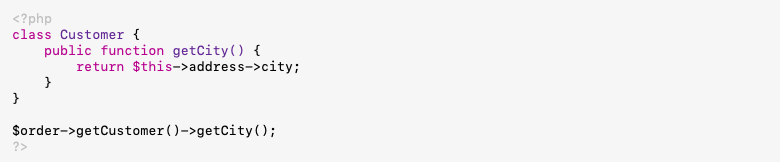
15. Man in middle
A class's methods simply call the methods of another class, acting as an intermediary and adding complexity.
Problematic code example:
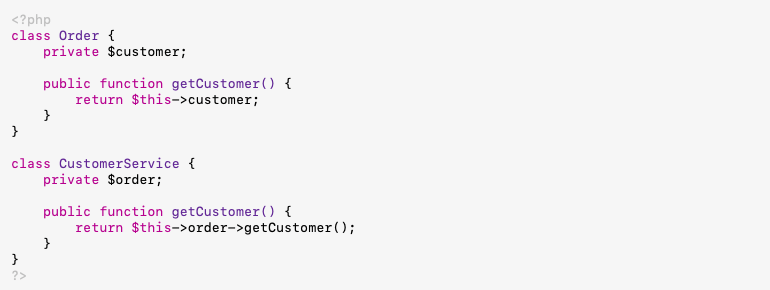
Solution example:
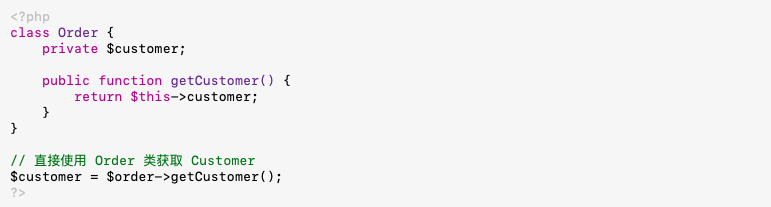
16. Array out of bounds
Accessing an array or list with an index that is out of its valid range.
Problematic code example:

Solution example:

17. Null pointer reference
Attempting to reference an uninitialized or freed object.
Problematic code example:

Solution example:

18. Memory leak
Memory allocated by the program is not released, causing continuous increase in memory usage.
Problematic code example:

Solution example:

19. Concurrency issues
Problems such as race conditions or deadlocks occur in multi-threaded or multi-process programs.
Problematic code example:

Solution example:
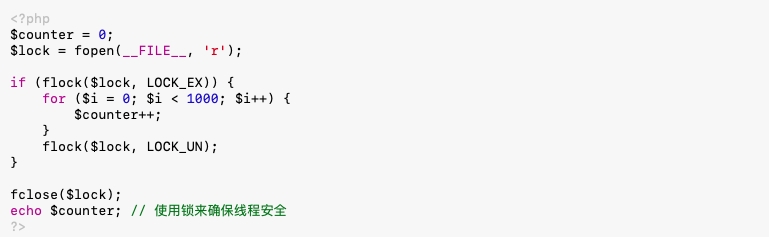
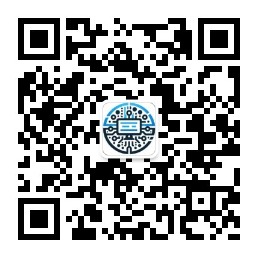
Follow us at WeChat to get more info